#Order Management
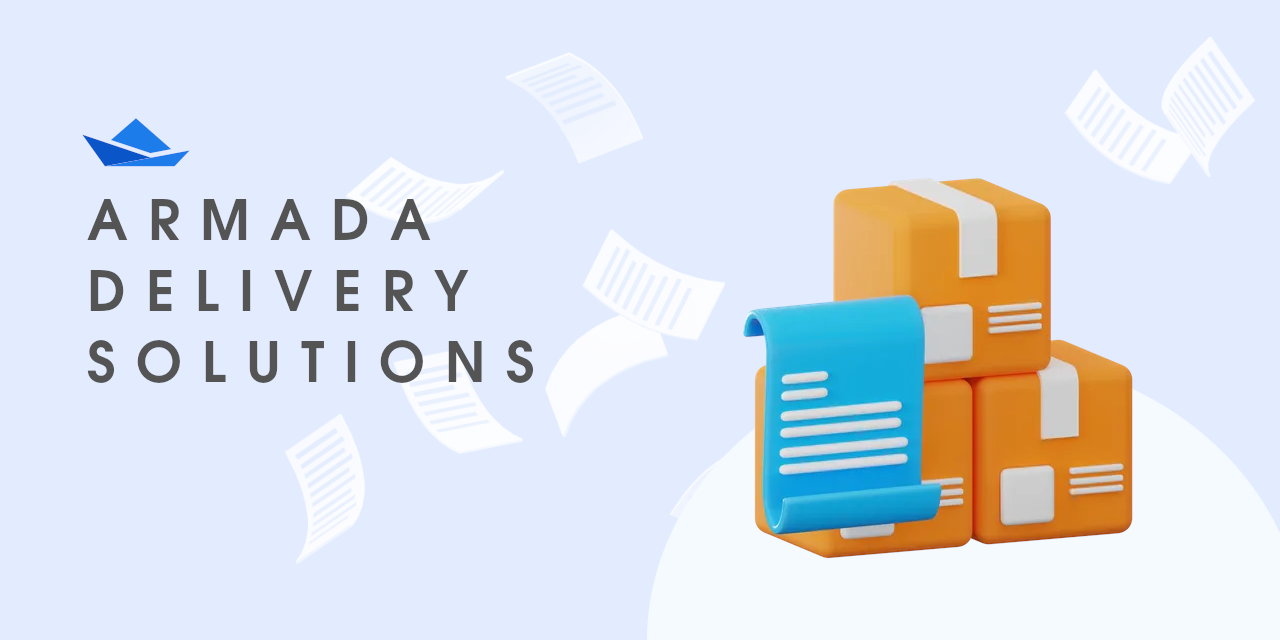
#Introduction
With our order management API, you can easily send orders to Armada drivers and receive details such as fees, estimates, tracking URLs, and more. Our service currently covers Kuwait, Bahrain, and Saudi Arabia (KSA).
#Note
To test your work on the sandbox or staging API, please refer to the Environments section for detailed instructions.
#Order Creation
To place an order, you will need to make an HTTP POST request to the following endpoint:
The body of the request should be in JSON format and include the following parameters:
platformName
: Refer to the delivery platform String - RequiredplatformData
: Contains the data required to create the delivery Object - RequiredorderId
: Order ID in your platform String - Requiredname
: Customer name String - Requiredphone
: Customer phone String - Requiredlocation
: Customer geo location address Object - Required if full address missinglatitude
: Floatlongitude
: Float
area
: Critical address component, must match our area spellings String - Required for Kuwait & Bahrain if location missingblock
: One of the address components String - Required for Kuwait & Bahrain if location missingstreet
: One of the address components String - Required for Kuwait & Bahrain if location missingbuildingNumber
: One of the address components String - Required for Kuwait & Bahrain if location missingfloor
: One of the address components String - Optionalapartment
: One of the address components String - Optionalinstructions
: Extra instructions for the driver String - OptionalshortAddress
: Short address version of the national address by SPL String - Required for KSA if location missingfirstLine
: Full normal address String - Required for KSA if location or shortAddress missingamount
: Order amount Number - Required if thepaymentType
iscash
scheduledAt
: Specify the desired start time for the order. This date must be later than the current time. If it's within 30 minutes from the current time, the order will be processed immediately ISODATE - OptionalpaymentType
: Payment type, Enum: should bepaid
orcash
String - Required
For the security, we recommend including a security key in the headers
of your order:
order-webhook-key
: Webhook key must be between 12-32 characters String - Recommend
When you receive an order update via your webhook, the order-webhook-key will be included in the headers as an Authorization key for security purposes. see webhooks
#Addressing
The addressing varies from one country to another. However, you can deliver your order using a unified method such as location
(latitude, longitude). If you prefer to provide a full address, please follow the format for each country.
#Kuwait π°πΌ Bahrain π§π
For Kuwait and Bahrain, We provide two ways in this order: location
or area
block
street
buildingNumber
.
#Saudi Arabia (KSA) πΈπ¦
For Saudi Arabia, We provide three ways in this order: location
or shortAddress
or firstLine
.
#Response Schema
After successful completion, our API will provide a JSON response containing the following parameters:
code
: Delivery ID within Armada StringdeliveryFee
: Cost of the delivery Numberamount
: Order amount set by the merchant NumbercustomerAddress
: Customer address generated by Armada based on platform data StringcustomerLocation
: Objectlatitude
: Latitude of the customer location Floatlongitude
: Longitude of the customer location Float
customerName
: Customer name StringcustomerPhone
: Customer phone number StringorderStatus
: Status of the order. (see webhooks status for more details) StringestimatedDistance
: Estimated distance from pickup to customer destination in meters NumberestimatedDuration
: Estimated duration from pickup to customer destination in seconds Numberdriver
: Driver data Objectname
: Driver name StringphoneNumber
: Driver phone String
trackingLink
: Link to a public page showing live delivery progress (give it to your client) URLqrCodeLink
: Link to the QR code for the driver to scan if not performed within the merchant dashboard URLorderCreatedAt
: Date of delivery creation ISO DATEcurrency
: Currency used for this delivery String
#Response Example
#Get Orders
To fetch an order, you will need to make an HTTP GET request to the following endpoint:
code
: Code returned by order creation.
#Request Example
(e.g code = E8E5653A05)
#Response Schema
For more details see Response Schema and Example of the previous section.
#Cancel Order
To cancel an order, you will need to make an HTTP POST request to the following endpoint:
code
: Code returned by order creation.
#Request Example
(e.g code = E8E5653A05)
#Response
- Upon successful cancellation, you will receive a
200
status code. - For failures, you will receive a
400
status code with the following body:
- Alternatively, you may receive a
404
status code for other failures.